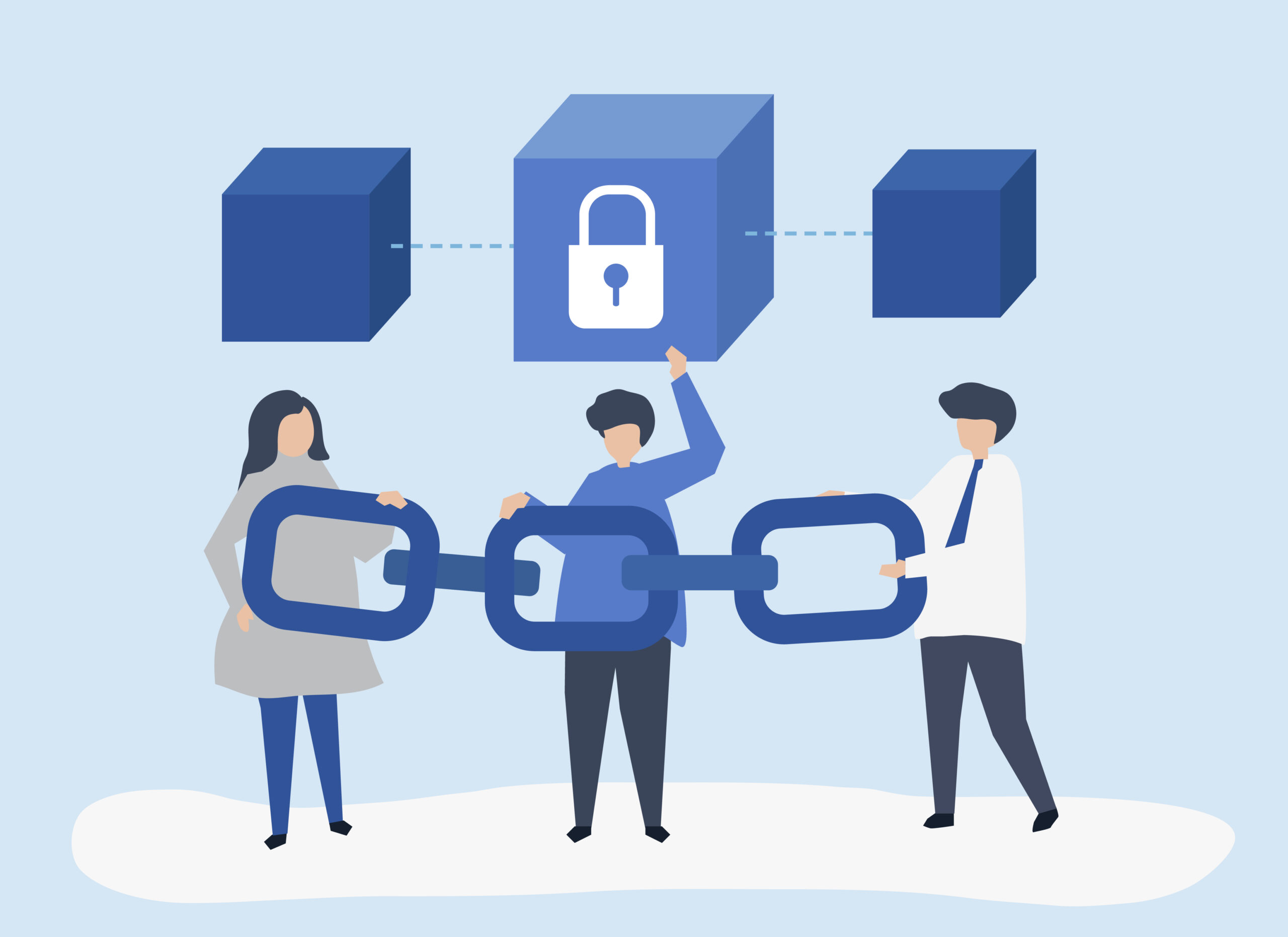
Network Steganography Code: Hiding Data in Plain Sight – Project For College Students
Network steganography is the art of concealing data within network protocols and traffic, allowing for covert communication and data transfer. In this blog post, we’ll explore the concept of network steganography and implement a simple program in Python to demonstrate how it works.
What is Network Steganography?
Steganography, derived from the Greek words “steganos” (covered) and “graphein” (writing), is the practice of hiding information within other information. In the context of networks, steganography involves concealing data within network protocols, such as TCP, UDP, IP, or even HTTP.
The primary goal of network steganography is to enable covert communication by hiding the very existence of the transmitted data. Unlike encryption, where the presence of encrypted data is evident, steganography aims to make the hidden data indistinguishable from regular network traffic.
Implementing Network Steganography in Python
To illustrate the concept of network steganography, we’ll create a simple program in Python that hides a secret message within the TCP headers of a network packet.
Here’s the code:
import socket
import struct
# Secret message to be hidden
secret_message = "This is a secret message!"
# Create a TCP socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# Convert the secret message to bytes
secret_bytes = secret_message.encode()
# Calculate the length of the secret message
secret_length = len(secret_bytes)
# Construct the TCP header with the secret message
# hidden in the sequence number field
tcp_header = struct.pack('!HHLLBBHHH', 0x0102, 0x0304, 0x05060708, 0x090a0b0c, 0x50, 0x01, 0x0d0e, 0x0f10, secret_length)
# Send the modified TCP header over the network
sock.sendto(tcp_header, ('192.168.1.100', 80))
# Close the socket
sock.close()
Let’s break down the code:
- We import the necessary modules:
socket
for network communication andstruct
for packing and unpacking data structures. - We define the secret message we want to hide:
"This is a secret message!"
. - We create a TCP socket using
socket.socket(socket.AF_INET, socket.SOCK_STREAM)
. - We convert the secret message to bytes using
secret_bytes = secret_message.encode()
. - We calculate the length of the secret message using
secret_length = len(secret_bytes)
. - We construct a modified TCP header using
struct.pack('!HHLLBBHHH', ...)
, where the secret message length is hidden in the sequence number field. - We send the modified TCP header over the network using
sock.sendto(tcp_header, ('192.168.1.100', 80))
, where'192.168.1.100'
is the destination IP address, and80
is the destination port. - Finally, we close the socket using
sock.close()
.
In this example, we’re hiding the length of the secret message within the sequence number field of the TCP header. The receiver can then extract the hidden data by analyzing the sequence number field and reconstructing the original message.
Limitations and Considerations
While network steganography can be a powerful tool for covert communication, it’s important to note that it has limitations and considerations:
- Bandwidth and Capacity: The amount of data that can be hidden within network protocols is generally limited, as modifying headers or payloads too extensively may raise suspicion or cause compatibility issues.
- Detection and Countermeasures: As steganography techniques become more widely known, detection and countermeasures also improve. Network administrators and security professionals may implement measures to detect and prevent steganography techniques.
- Legal and Ethical Considerations: Network steganography can be used for both legitimate and malicious purposes. It’s crucial to ensure that any use of steganography complies with applicable laws and ethical guidelines.
Additional Information on How To Run Above Code:
To run the provided Python code, you’ll need to save it in a Python file (e.g., network_steganography.py
) and then execute it from the command line or a Python integrated development environment (IDE).
Here are the steps to run the code:
- Open a text editor and create a new file
- Open a text editor of your choice (e.g., Sublime Text, Visual Studio Code, Notepad++).
- Create a new file and save it with a
.py
extension (e.g.,network_steganography.py
).
- Copy and paste the code into the file
- Copy the provided code and paste it into the newly created Python file.
- Save the file
- Save the file after pasting the code.
- Open a terminal or command prompt
- On Windows, you can open the Command Prompt by pressing the
Win + R
keys, typingcmd
, and pressing Enter. - On macOS or Linux, you can open the terminal application.
- On Windows, you can open the Command Prompt by pressing the
- Navigate to the directory where the Python file is saved
- Use the
cd
command to navigate to the directory where you saved thenetwork_steganography.py
file. - For example, if the file is saved on your Desktop, you can navigate to it by typing
cd Desktop
(on Windows) orcd ~/Desktop
(on macOS/Linux).
- Use the
- Run the Python file
- Once you’re in the correct directory, you can run the Python file by typing
python network_steganography.py
(on Windows) orpython3 network_steganography.py
(on macOS/Linux) and pressing Enter.
- Once you’re in the correct directory, you can run the Python file by typing
The code will execute, and it will attempt to send the modified TCP header with the hidden secret message to the IP address 192.168.1.100
on port 80
.
Note that this code is for demonstration purposes only and will only work if there is a recipient listening on the specified IP address and port. Additionally, you may need to run the script with administrative privileges (e.g., sudo
on macOS/Linux) if you encounter permission issues.
It’s also important to note that using network steganography techniques for malicious purposes or without proper authorization may be illegal in some jurisdictions. Always ensure that you are using these techniques responsibly and within legal and ethical boundaries.
Conclusion
Network steganography is a fascinating field that allows for covert communication by hiding data within network protocols and traffic. While the example provided in this blog post is relatively simple, it demonstrates the basic concept of network steganography. However, it’s important to remember that steganography should be used responsibly and in compliance with legal and ethical guidelines.