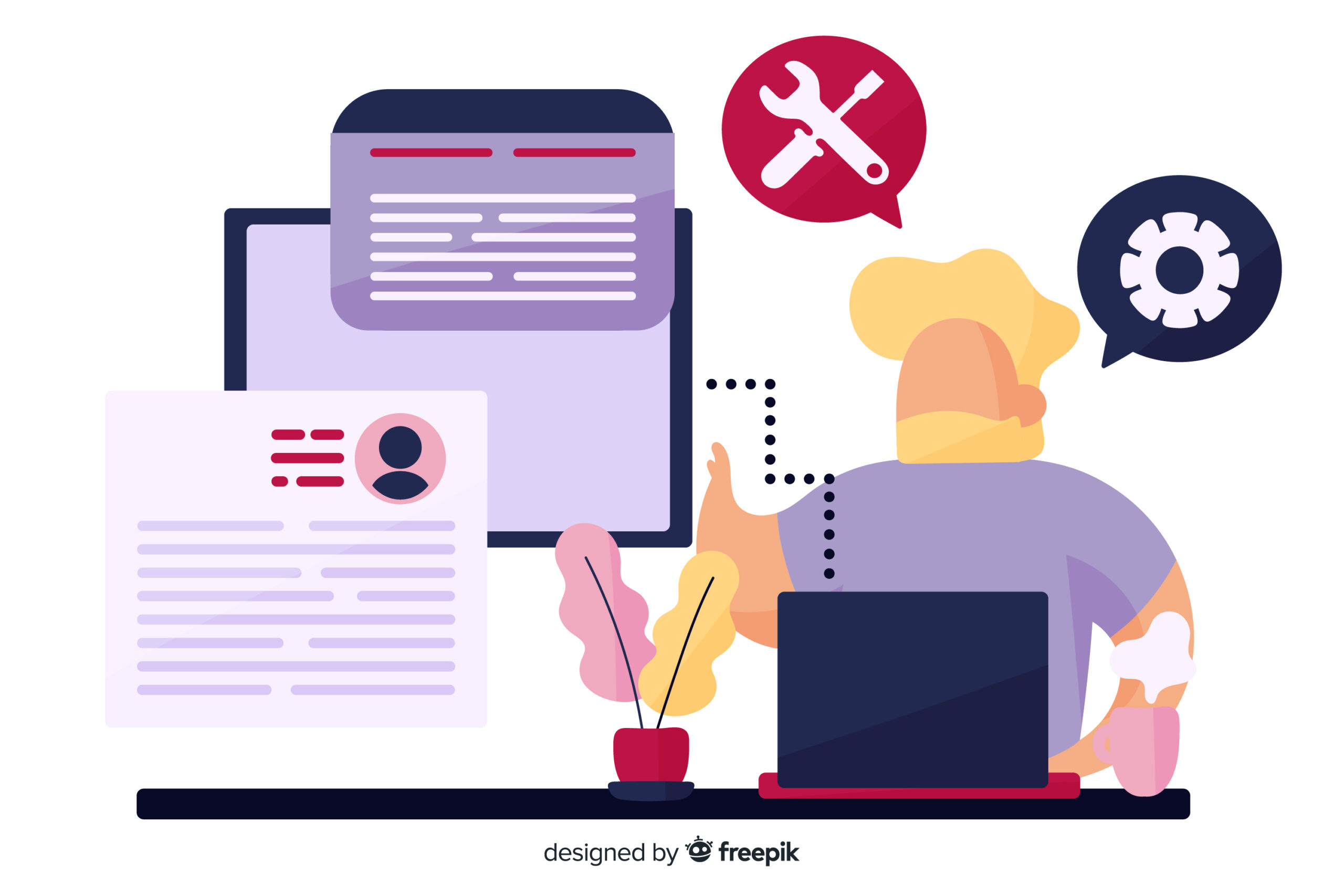
Debugging code is an essential skill for any programmer. No matter how experienced you are, errors and bugs are an inevitable part of software development. Whether you’re a seasoned developer or just starting your coding journey, understanding effective debugging techniques can save you a lot of time and frustration. In this comprehensive guide, we’ll explore the steps to debugging code and how to efficiently find and fix errors in your programs.
1. Understanding the Basics of Debugging
Before diving into the specific steps of debugging, it’s important to grasp the fundamental concepts and techniques that will serve as the foundation for your debugging process.
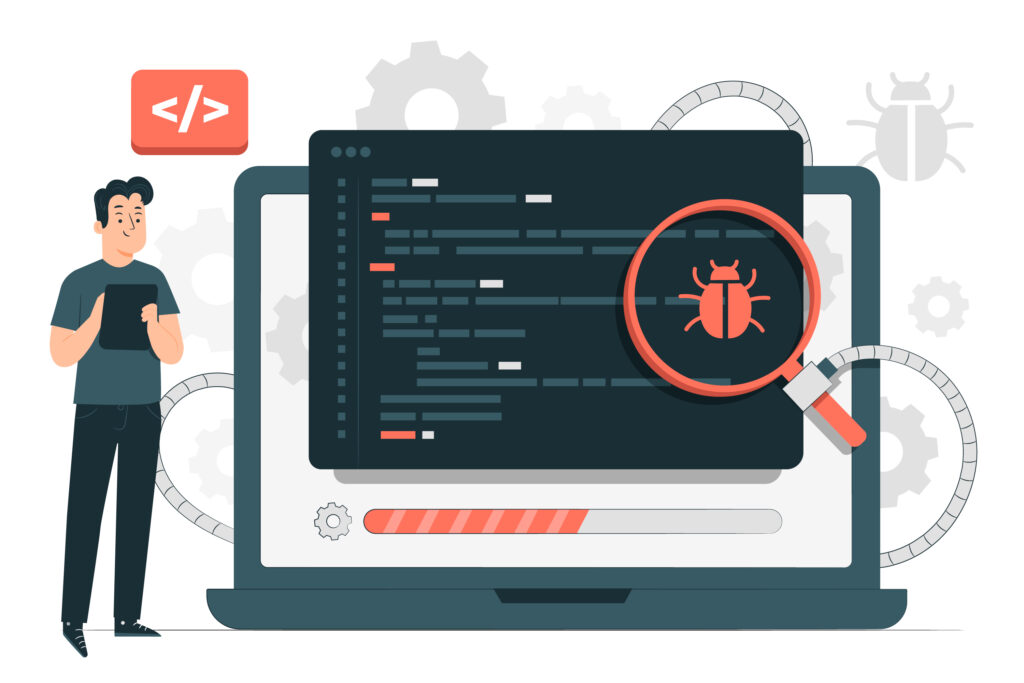
1.1. Know Your Tools
The first step in efficient debugging is to familiarize yourself with the debugging tools available in your chosen programming environment. Most integrated development environments (IDEs) offer a range of debugging features such as breakpoints, watchlists, and call stacks. Learning how to use these tools effectively is essential for a smooth debugging experience.
1.2. Reproduce the Bug
To fix an error, you first need to understand and reproduce it. This involves identifying the specific inputs or conditions that trigger the error. Creating a minimal and isolated test case that replicates the problem can be immensely helpful. With a reproducible scenario in hand, you can proceed to analyze the error more effectively.
1.3. Read the Error Messages
Error messages are your friends in the debugging process. They provide valuable information about what went wrong and where the issue might be. Make it a habit to read error messages carefully. They often contain error codes, line numbers, and descriptions that can guide you in the right direction.
1.4. Break the Problem Down
Complex issues are often composed of several smaller problems. Break down your code into smaller components and test them individually. This can help you identify which part of your code is causing the error, making it easier to isolate and fix the issue.
2. The Debugging Process
Now that you have a solid foundation in debugging principles, let’s dive into the step-by-step debugging process.
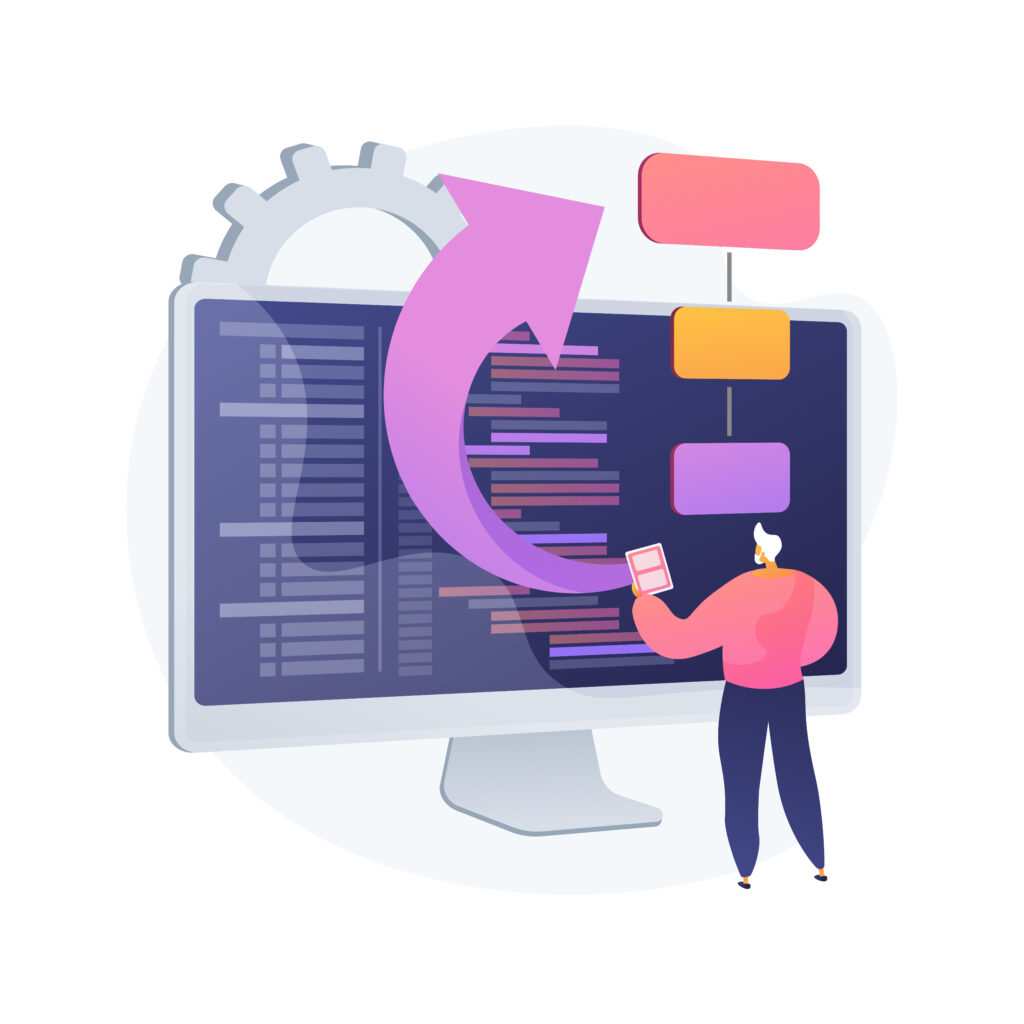
2.1. Step 1: Identify the Problem
The first step in debugging is to pinpoint the problem. Once you’ve reproduced the bug and read any relevant error messages, take a close look at the code where the error occurs. Analyze the code logic and variables to determine what’s going wrong. This may involve inspecting the values of variables, checking conditions, and evaluating the flow of the program.
2.2. Step 2: Add Debugging Statements
One of the most effective ways to understand the flow of your code is by adding debugging statements. These are temporary print statements or log entries that display the values of variables, execution points, or any other relevant information. By strategically placing these statements in your code, you can track the execution and identify the exact location of the error.
def calculate_average(numbers): total = 0 count = 0 for num in numbers: total += num count += 1 print(f”Total: {total}, Count: {count}”) # Debugging statement return total / count |
2.3. Step 3: Use Breakpoints
Breakpoints are a powerful debugging tool that allows you to pause the execution of your program at a specific line of code. While the program is paused, you can inspect variables, evaluate expressions, and step through the code one line at a time. This feature is particularly useful for analyzing the state of your program at a specific point and understanding how it reaches the error.
2.4. Step 4: Review Your Code
Sometimes, the error might not be in the immediate vicinity of where it’s reported. It could be the result of a logic error or an oversight in another part of your code. Take a step back and review the entire codebase. Check for potential issues, such as incorrect variable names, missing or incorrect function calls, or issues with imported modules.
2.5. Step 5: Test Hypotheses
During the debugging process, you may develop hypotheses about the root cause of the error. Test these hypotheses by making changes to the code and observing the effects. Be systematic in your approach, and keep track of what you’ve tested and the results you’ve obtained.
2.6. Step 6: Seek Help
Debugging can be a challenging and time-consuming task, and it’s perfectly normal to encounter problems that leave you stumped. Don’t hesitate to seek help from colleagues, online forums, or documentation. Sharing your problem with others can provide fresh perspectives and solutions you might not have considered.
3. Preventing Future Bugs
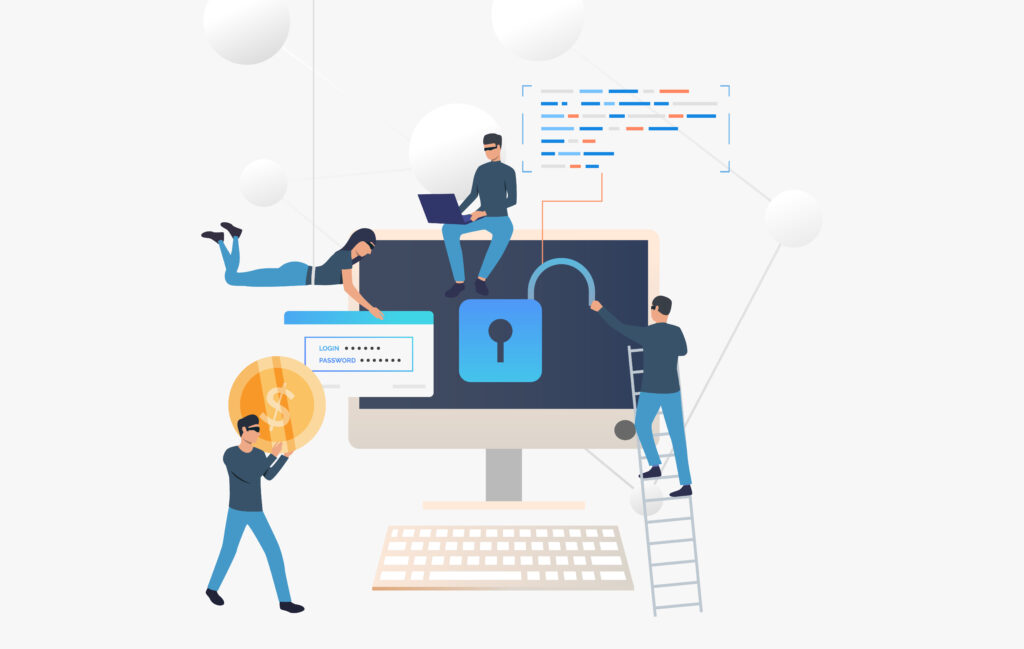
While debugging is an essential skill, it’s even better to write code that is less prone to errors in the first place. Here are some strategies to help you prevent bugs from creeping into your code.
3.1. Code Reviews
Code reviews involve having another programmer review your code before it’s merged into the main codebase. This process helps catch errors, improve code quality, and share knowledge among team members. By adopting a code review process, you can significantly reduce the number of bugs that make their way into your software.
3.2. Automated Testing
Automated testing involves writing scripts or test cases that verify the correctness of your code automatically. Unit tests, integration tests, and regression tests can catch bugs early in the development process, allowing you to address them before they become more challenging and expensive to fix.
3.3. Version Control
Version control systems, such as Git, enable you to track changes to your code and provide the ability to roll back to previous versions when issues arise. By using version control, you can easily identify the point in time when a bug was introduced and work to fix it from there.
3.4. Coding Standards and Guidelines
Following coding standards and best practices can help you write code that is easier to read and less error-prone. Consistent indentation, meaningful variable names, and well-documented code can all contribute to fewer bugs and more maintainable software.
3.5. Continuous Learning
Technology and programming languages evolve, and new tools and practices are constantly emerging. Staying up to date with the latest developments in your field can help you avoid common pitfalls and take advantage of modern debugging techniques.
Conclusion
Debugging is an essential part of software development, and becoming proficient at it takes time and practice. By understanding the basics of debugging, following a systematic debugging process, and taking steps to prevent future bugs, you can become a more efficient and effective programmer. Remember that debugging is not a sign of failure but an opportunity to improve your skills and produce higher-quality code. So, the next time you encounter a bug in your code, don’t be discouraged. Follow these steps, and you’ll be on your way to finding and fixing errors efficiently. Happy debugging!